500
|
How can I filter items for dates before a specified date
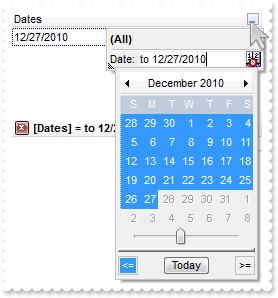
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Dates")));
var_Column->PutSortType(EXTREELib::SortDate);
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_TRUE);
var_Column->PutDisplayFilterDate(VARIANT_TRUE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowFocusItem | EXTREELib::exNoItems));
var_Column->PutFilter(L"to 12/27/2010");
var_Column->PutFilterType(EXTREELib::exDate);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2010,12,27,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,28,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,29,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,30,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,31,0,00,00).operator DATE());
spTree1->ApplyFilter();
spTree1->EndUpdate();
|
499
|
Is it possible to filter dates
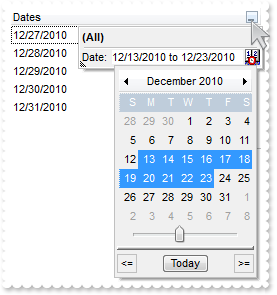
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Dates")));
var_Column->PutSortType(EXTREELib::SortDate);
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_TRUE);
var_Column->PutDisplayFilterDate(VARIANT_TRUE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowFocusItem | EXTREELib::exNoItems));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2010,12,27,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,28,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,29,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,30,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2010,12,31,0,00,00).operator DATE());
spTree1->EndUpdate();
|
498
|
Is it possible to change the Exclude field name to something different, in the drop down filter window
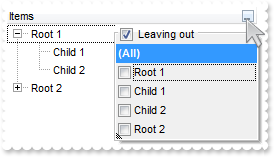
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->PutDescription(EXTREELib::exFilterBarExclude,L"Leaving out");
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowExclude | EXTREELib::exShowFocusItem | EXTREELib::exShowCheckBox));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
spTree1->EndUpdate();
|
497
|
How can I display the Exclude field in the drop down filter window
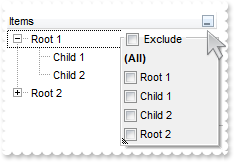
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowExclude | EXTREELib::exShowFocusItem | EXTREELib::exShowCheckBox));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
spTree1->EndUpdate();
|
496
|
Is it possible to show and ensure the focused item from the control, in the drop down filter window
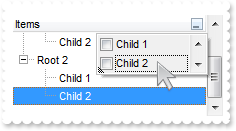
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowFocusItem | EXTREELib::exShowCheckBox));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->PutSelectItem(var_Items->InsertItem(h,vtMissing,"Child 2"),VARIANT_TRUE);
var_Items->PutExpandItem(h,VARIANT_TRUE);
spTree1->EndUpdate();
|
495
|
Is it possible to show only blanks items with no listed items from the control
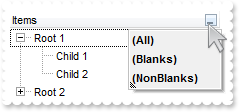
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowBlanks | EXTREELib::exNoItems));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->EndUpdate();
|
494
|
How can I include the blanks items in the drop down filter window
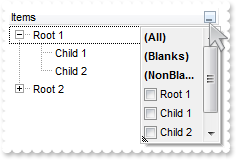
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::FilterListEnum(EXTREELib::exShowBlanks | EXTREELib::exShowCheckBox));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->EndUpdate();
|
493
|
How can I select multiple items in the drop down filter window, using check-boxes
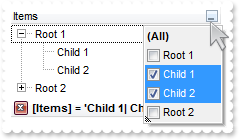
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::exShowCheckBox);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->EndUpdate();
|
492
|
Is it possible to allow a single item being selected in the drop down filter window
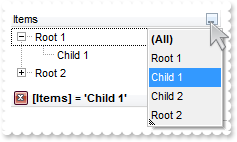
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXTREELib::exSingleSel);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->EndUpdate();
|
491
|
How can I display no (All) item in the drop down filter window
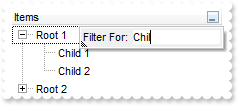
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->PutDescription(EXTREELib::exFilterBarAll,L"");
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_TRUE);
var_Column->PutFilterList(EXTREELib::exNoItems);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->EndUpdate();
|
490
|
Is it possible to display no items in the drop down filter window, so only the pattern is visible
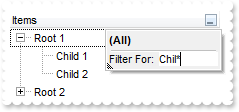
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Items")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_TRUE);
var_Column->PutFilterList(EXTREELib::exNoItems);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->EndUpdate();
|
489
|
How do I hide the selection

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSelForeColor(spTree1->GetForeColor());
spTree1->PutSelBackColor(spTree1->GetBackColor());
spTree1->PutShowFocusRect(VARIANT_FALSE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(var_Columns->Add(L"Format")));
var_Column->PutFormatColumn(_bstr_t("type(value) in (0,1) ? 'null' : ( dbl(value)<0 ? '<fgcolor=FF0000>'+ (value format '2|.|3|,|1' ) : (dbl(value)>0 ? '<fgcolor=00") +
"00FF>+'+(value format '2|.|3|,' ): '0.00') )");
var_Column->PutDef(EXTREELib::exCellCaptionFormat,long(1));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(10));
var_Items->AddItem(long(-8));
spTree1->EndUpdate();
|
488
|
How do I access the cells, or how do I get the values in the columns
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"C1");
var_Columns->Add(L"C2");
var_Columns->Add(L"C3");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Item 1");
var_Items->PutCellCaption(h,long(1),"SubItem 1.1");
var_Items->PutCellCaption(h,long(2),"SubItem 1.2");
OutputDebugStringW( _bstr_t(var_Items->GetCellCaption(h,long(0))) );
|
487
|
Is it possible to load child items when clicking the +/- button
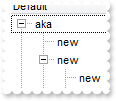
// BeforeExpandItem event - Fired before an item is about to be expanded (collapsed).
void OnBeforeExpandItemTree1(long Item,VARIANT FAR* Cancel)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->InsertItem(Item,vtMissing,"new");
var_Items->PutItemHasChildren(var_Items->InsertItem(Item,vtMissing,"new"),VARIANT_TRUE);
var_Items->InsertItem(Item,vtMissing,"new");
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutItemHasChildren(var_Items->AddItem("aka"),VARIANT_TRUE);
var_Items->AddItem("next item");
|
486
|
How can I change the check-boxes appearance
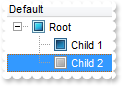
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Default")));
var_Column->PutDef(EXTREELib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutPartialCheck(VARIANT_TRUE);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
EXTREELib::IAppearancePtr var_Appearance = spTree1->GetVisualAppearance();
var_Appearance->Add(1,"XP:Button 3 12");
var_Appearance->Add(2,"XP:Button 3 11");
var_Appearance->Add(3,"XP:Button 3 10");
spTree1->PutCheckImage(EXTREELib::Unchecked,16777216);
spTree1->PutCheckImage(EXTREELib::Checked,33554432);
spTree1->PutCheckImage(EXTREELib::PartialChecked,50331648);
|
485
|
How can I initiate the OLE Drag and Drop support
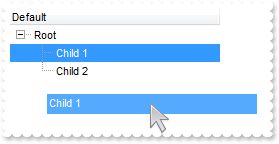
// OLEStartDrag event - Occurs when the OLEDrag method is called.
void OnOLEStartDragTree1(LPDISPATCH Data,long FAR* AllowedEffects)
{
// Data.SetData("data to drag")
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
AllowedEffects = 1;
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutOLEDropMode(EXTREELib::exOLEDropManual);
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
484
|
How can I apply the same ConditionalFormat on more than 1(one) column (multiple columns and not on item)
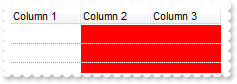
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
EXTREELib::IConditionalFormatPtr var_ConditionalFormat = spTree1->GetConditionalFormats()->Add(L"1","K1");
var_ConditionalFormat->PutBackColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXTREELib::FormatApplyToEnum(0x1));
EXTREELib::IConditionalFormatPtr var_ConditionalFormat1 = spTree1->GetConditionalFormats()->Add(L"1","K2");
var_ConditionalFormat1->PutBackColor(RGB(255,0,0));
var_ConditionalFormat1->PutApplyTo(EXTREELib::FormatApplyToEnum(0x2));
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutDrawGridLines(EXTREELib::exRowLines);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Column 1");
var_Columns->Add(L"Column 2");
var_Columns->Add(L"Column 3");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(vtMissing);
var_Items->AddItem(vtMissing);
var_Items->AddItem(vtMissing);
spTree1->EndUpdate();
|
483
|
Is it possible to display empty strings for 0 values
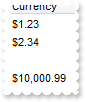
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Currency")))->PutFormatColumn(L"dbl(value) ? currency(dbl(value)) : ``");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(double(1.23));
var_Items->AddItem(double(2.34));
var_Items->AddItem(long(0));
var_Items->AddItem(double(10000.99));
|
482
|
Is it possible to display empty strings for 0 values
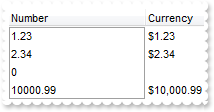
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Number");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Currency")))->PutComputedField(L"%0 ? currency(%0) : ``");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(double(1.23));
var_Items->AddItem(double(2.34));
var_Items->AddItem(long(0));
var_Items->AddItem(double(10000.99));
|
481
|
How can I get the list of items as they are displayed
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutBackColorAlternate(RGB(240,240,240));
spTree1->GetColumns()->Add(L"Names");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("Mantel");
var_Items->AddItem("Mechanik");
var_Items->AddItem("Motor");
var_Items->AddItem("Murks");
var_Items->AddItem("Märchen");
var_Items->AddItem("Möhren");
var_Items->AddItem("Mühle");
spTree1->GetColumns()->GetItem(long(0))->PutSortOrder(EXTREELib::SortAscending);
spTree1->EndUpdate();
OutputDebugStringW( _bstr_t(spTree1->GetItems(long(1))) );
|
480
|
Is posible to reduce the size of the picture to be shown in the column's caption
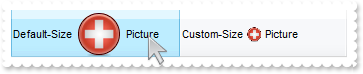
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutHTMLPicture(L"pic1","c:\\exontrol\\images\\zipdisk.gif");
spTree1->PutHeaderHeight(48);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"DefaultSize")))->PutHTMLCaption(L"Default-Size <img>pic1</img> Picture");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"CustomSize")))->PutHTMLCaption(L"Custom-Size <img>pic1:16</img> Picture");
spTree1->EndUpdate();
|
479
|
How can I change the color, font, bold etc for the items/cells in the same column or for the entire column
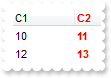
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
EXTREELib::IConditionalFormatPtr var_ConditionalFormat = spTree1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutBold(VARIANT_TRUE);
var_ConditionalFormat->PutForeColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXTREELib::FormatApplyToEnum(0x1));
spTree1->GetColumns()->Add(L"C1");
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"C2")));
var_Column->PutHeaderBold(VARIANT_TRUE);
var_Column->PutHTMLCaption(L"<fgcolor=FF0000>C2");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(10)),long(1),long(11));
var_Items->PutCellCaption(var_Items->AddItem(long(12)),long(1),long(13));
spTree1->EndUpdate();
|
478
|
The item is not getting selected when clicking the cell's checkbox. What should I do
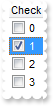
// CellStateChanged event - Fired after cell's state has been changed.
void OnCellStateChangedTree1(long Item,long ColIndex)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetItems()->PutSelectItem(Item,VARIANT_TRUE);
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Check")))->PutDef(EXTREELib::exCellHasCheckBox,VARIANT_TRUE);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
var_Items->AddItem(long(3));
|
477
|
Is it possible to limit the height of the item while resizing
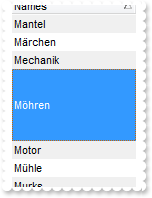
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemTree1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetItems()->PutItemMinHeight(Item,18);
spTree1->GetItems()->PutItemMaxHeight(Item,72);
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutItemsAllowSizing(EXTREELib::exResizeItem);
spTree1->PutScrollBySingleLine(VARIANT_FALSE);
spTree1->PutBackColorAlternate(RGB(240,240,240));
spTree1->GetColumns()->Add(L"Names");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("Mantel");
var_Items->AddItem("Mechanik");
var_Items->AddItem("Motor");
var_Items->AddItem("Murks");
var_Items->AddItem("Märchen");
var_Items->AddItem("Möhren");
var_Items->AddItem("Mühle");
spTree1->GetColumns()->GetItem(long(0))->PutSortOrder(EXTREELib::SortAscending);
spTree1->EndUpdate();
|
476
|
Is it possible to copy the hierarchy of the control using the GetItems method
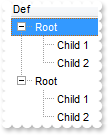
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Def");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
spTree1->PutItems(spTree1->GetItems(long(-1)),vtMissing);
|
475
|
How can I show the child items with no identation
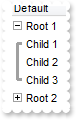
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exGroupLinesOutside);
spTree1->PutIndent(12);
spTree1->PutHasLines(EXTREELib::exThinLine);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
|
474
|
Is there other ways of showing the hierarchy lines (exGroupLinesAtRoot)
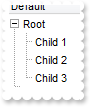
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exGroupLinesAtRoot);
spTree1->PutIndent(12);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
473
|
Is there other ways of showing the hierarchy lines (exGroupLinesOutside)
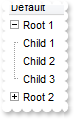
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exGroupLinesOutside);
spTree1->PutIndent(12);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
|
472
|
Is there other ways of showing the hierarchy lines (exGroupLinesInsideLeaf)
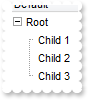
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exGroupLinesInsideLeaf);
spTree1->PutIndent(12);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
471
|
Is there other ways of showing the hierarchy lines (exGroupLinesInside)
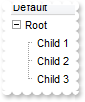
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exGroupLinesInside);
spTree1->PutIndent(12);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
470
|
Is there other ways of showing the hierarchy lines (exGroupLines)
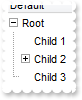
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutLinesAtRoot(EXTREELib::exGroupLines);
spTree1->PutIndent(12);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(var_Items->InsertItem(h,vtMissing,"Child 2"),vtMissing,"SubChild 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
469
|
Does your control supports multiple lines tooltip
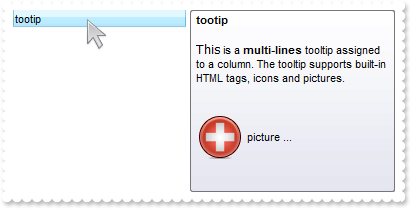
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutHTMLPicture(L"pic1","c:\\exontrol\\images\\zipdisk.gif");
spTree1->PutToolTipDelay(1);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"tootip")))->PutToolTip(_bstr_t("<br><font Tahoma;10>This</font> is a <b>multi-lines</b> tooltip assigned to a column. The tooltip supports built-in HTML tags, ") +
"icons and pictures.<br><br><br><img>pic1</img> picture ... <br><br>");
|
468
|
How can I prevent highlighting the column from the cursor - point

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetVisualAppearance()->Add(1,_bstr_t("gBFLBCJwBAEHhEJAEGg4BI0IQAAYAQGKIYBkAKBQAGaAoDDUOQzQwAAxDKKUEwsACEIrjKCYVgOHYYRrIMYgBCMJhLEoaZLhEZRQiqDYtRDFQBSDDcPw/EaRZohGaYJ") +
"gEgI=");
spTree1->PutBackground(EXTREELib::exCursorHoverColumn,0x1000000);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"S")))->PutWidth(32);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Level 1")))->PutLevelKey(long(1));
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Level 2")))->PutLevelKey(long(1));
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Level 3")))->PutLevelKey(long(1));
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"E1")))->PutWidth(32);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"E2")))->PutWidth(32);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"E3")))->PutWidth(32);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"E4")))->PutWidth(32);
|
467
|
Is there any option to show the tooltip programmatically
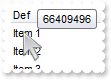
// MouseMove event - Occurs when the user moves the mouse.
void OnMouseMoveTree1(short Button,short Shift,long X,long Y)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->ShowToolTip(_bstr_t(spTree1->GetItemFromPoint(-1,-1,c,hit)),"","8","8",vtMissing);
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->GetColumns()->Add(L"Def");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
spTree1->EndUpdate();
|
466
|
Is it possible to prevent changing the rows/items colors by selection
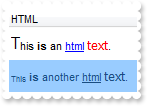
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutSelBackMode(EXTREELib::exTransparent);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"HTML")))->PutDef(EXTREELib::exCellCaptionFormat,long(1));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("<font ;12>T</font>his <b>is</b> an <a>html</a> <font Tahoma><fgcolor=FF0000>text</fgcolor></font>.");
var_Items->PutSelectItem(var_Items->AddItem("<font ;6>This</font> <b>is</b> another <a>html</a> <font Tahoma><fgcolor=FF0000>text</fgcolor></font>."),VARIANT_TRUE);
|
465
|
Is it possible to specify the cell's value but still want to display some formatted text instead the value
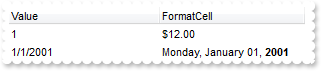
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->GetColumns()->Add(L"Value");
spTree1->GetColumns()->Add(L"FormatCell");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem(long(1));
var_Items->PutCellCaption(h,long(1),long(12));
var_Items->PutFormatCell(h,long(1),L"currency(value)");
h = var_Items->AddItem(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->PutCellCaptionFormat(h,long(1),EXTREELib::exHTML);
var_Items->PutFormatCell(h,long(1),L"longdate(value) replace '2001' with '<b>2001</b>'");
spTree1->EndUpdate();
|
464
|
How can I simulate displaying groups
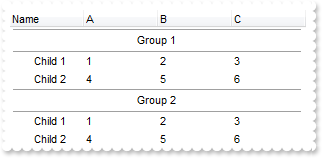
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutHasLines(EXTREELib::exNoLine);
spTree1->PutScrollBySingleLine(VARIANT_TRUE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Name");
var_Columns->Add(L"A");
var_Columns->Add(L"B");
var_Columns->Add(L"C");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Group 1");
var_Items->PutCellHAlignment(h,long(0),EXTREELib::CenterAlignment);
var_Items->PutItemDivider(h,0);
var_Items->PutItemDividerLineAlignment(h,EXTREELib::DividerBoth);
var_Items->PutItemHeight(h,24);
var_Items->PutSortableItem(h,VARIANT_FALSE);
long h1 = var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->PutCellCaption(h1,long(1),long(1));
var_Items->PutCellCaption(h1,long(2),long(2));
var_Items->PutCellCaption(h1,long(3),long(3));
h1 = var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutCellCaption(h1,long(1),long(4));
var_Items->PutCellCaption(h1,long(2),long(5));
var_Items->PutCellCaption(h1,long(3),long(6));
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Group 2");
var_Items->PutCellHAlignment(h,long(0),EXTREELib::CenterAlignment);
var_Items->PutItemDivider(h,0);
var_Items->PutItemDividerLineAlignment(h,EXTREELib::DividerBoth);
var_Items->PutItemHeight(h,24);
var_Items->PutSortableItem(h,VARIANT_FALSE);
h1 = var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->PutCellCaption(h1,long(1),long(1));
var_Items->PutCellCaption(h1,long(2),long(2));
var_Items->PutCellCaption(h1,long(3),long(3));
h1 = var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutCellCaption(h1,long(1),long(4));
var_Items->PutCellCaption(h1,long(2),long(5));
var_Items->PutCellCaption(h1,long(3),long(6));
var_Items->PutExpandItem(h,VARIANT_TRUE);
spTree1->EndUpdate();
|
463
|
How can I collapse all items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Items");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(0,VARIANT_FALSE);
spTree1->EndUpdate();
|
462
|
How can I expand all items
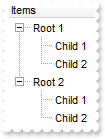
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Items");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(0,VARIANT_TRUE);
spTree1->EndUpdate();
|
461
|
Can I use PNG images to display pictures in the control
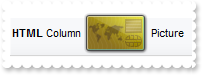
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->PutHTMLPicture(L"pic1","c:\\exontrol\\images\\card.png");
spTree1->PutHeaderHeight(48);
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"ColumnName")))->PutHTMLCaption(L"<b>HTML</b> Column <img>pic1</img> Picture");
|
460
|
Is it possible to move an item from a parent to another

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Items");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("A");
var_Items->AddItem("B");
var_Items->InsertItem(var_Items->AddItem("C"),"","D");
var_Items->SetParent(var_Items->GetFindItem("D",long(0),vtMissing),var_Items->GetFindItem("A",long(0),vtMissing));
spTree1->EndUpdate();
|
459
|
How can I change the identation for an item
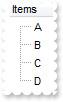
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Items");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("A");
var_Items->AddItem("B");
var_Items->InsertItem(var_Items->AddItem("C"),"","D");
var_Items->SetParent(var_Items->GetFindItem("D",long(0),vtMissing),0);
spTree1->EndUpdate();
|
458
|
How can I filter programatically using more columns
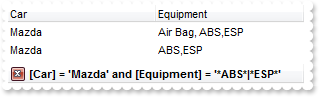
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Car");
var_Columns->Add(L"Equipment");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem("Mazda"),long(1),"Air Bag");
var_Items->PutCellCaption(var_Items->AddItem("Toyota"),long(1),"Air Bag,Air condition");
var_Items->PutCellCaption(var_Items->AddItem("Ford"),long(1),"Air condition");
var_Items->PutCellCaption(var_Items->AddItem("Nissan"),long(1),"Air Bag,ABS,ESP");
var_Items->PutCellCaption(var_Items->AddItem("Mazda"),long(1),"Air Bag, ABS,ESP");
var_Items->PutCellCaption(var_Items->AddItem("Mazda"),long(1),"ABS,ESP");
EXTREELib::IColumnPtr var_Column = spTree1->GetColumns()->GetItem("Car");
var_Column->PutFilterType(EXTREELib::exFilter);
var_Column->PutFilter(L"Mazda");
EXTREELib::IColumnPtr var_Column1 = spTree1->GetColumns()->GetItem("Equipment");
var_Column1->PutFilterType(EXTREELib::exPattern);
var_Column1->PutFilter(L"*ABS*|*ESP*");
spTree1->ApplyFilter();
spTree1->EndUpdate();
|
457
|
Is it possible to colour a particular column, I mean the cell's foreground color
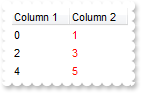
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
EXTREELib::IConditionalFormatPtr var_ConditionalFormat = spTree1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutForeColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXTREELib::FormatApplyToEnum(0x1));
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Column 1");
var_Columns->Add(L"Column 2");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(0)),long(1),long(1));
var_Items->PutCellCaption(var_Items->AddItem(long(2)),long(1),long(3));
var_Items->PutCellCaption(var_Items->AddItem(long(4)),long(1),long(5));
spTree1->EndUpdate();
|
456
|
Is it possible to colour a particular column for specified values
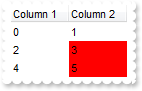
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
EXTREELib::IConditionalFormatPtr var_ConditionalFormat = spTree1->GetConditionalFormats()->Add(L"int(%1) in (3,4,5)",vtMissing);
var_ConditionalFormat->PutBackColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXTREELib::FormatApplyToEnum(0x1));
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Column 1");
var_Columns->Add(L"Column 2");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(0)),long(1),long(1));
var_Items->PutCellCaption(var_Items->AddItem(long(2)),long(1),long(3));
var_Items->PutCellCaption(var_Items->AddItem(long(4)),long(1),long(5));
spTree1->EndUpdate();
|
455
|
Is it possible to colour a particular column
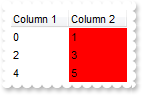
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Column 1");
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Column 2")))->PutDef(EXTREELib::exCellBackColor,long(255));
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(0)),long(1),long(1));
var_Items->PutCellCaption(var_Items->AddItem(long(2)),long(1),long(3));
var_Items->PutCellCaption(var_Items->AddItem(long(4)),long(1),long(5));
spTree1->EndUpdate();
|
454
|
How do i get all the children items that are under a certain parent Item handle
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"P");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
EXTREELib::IItemsPtr var_Items1 = spTree1->GetItems();
long hChild = var_Items1->GetItemChild(var_Items1->GetFirstVisibleItem());
OutputDebugStringW( _bstr_t(var_Items1->GetCellCaption(hChild,long(0))) );
OutputDebugStringW( _bstr_t(var_Items1->GetCellCaption(var_Items1->GetNextSiblingItem(hChild),long(0))) );
spTree1->EndUpdate();
|
453
|
How can I get the caption of focused item
// SelectionChanged event - Fired after a new item has been selected.
void OnSelectionChangedTree1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
OutputDebugStringW( L"Handle" );
OutputDebugStringW( _bstr_t(var_Items->GetFocusItem()) );
OutputDebugStringW( L"Caption" );
OutputDebugStringW( _bstr_t(var_Items->GetCellCaption(var_Items->GetFocusItem(),long(0))) );
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Items");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("R1");
var_Items->InsertItem(h,vtMissing,"Cell 1.1");
var_Items->InsertItem(h,vtMissing,"Cell 1.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("R2");
var_Items->InsertItem(h,vtMissing,"Cell 2.1");
var_Items->InsertItem(h,vtMissing,"Cell 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spTree1->EndUpdate();
|
452
|
How can I get the caption of selected item
// SelectionChanged event - Fired after a new item has been selected.
void OnSelectionChangedTree1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
OutputDebugStringW( L"Handle" );
OutputDebugStringW( _bstr_t(var_Items->GetSelectedItem(0)) );
OutputDebugStringW( L"Caption" );
OutputDebugStringW( _bstr_t(var_Items->GetCellCaption(var_Items->GetSelectedItem(0),long(0))) );
}
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
spTree1->GetColumns()->Add(L"Items");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("R1");
var_Items->InsertItem(h,vtMissing,"Cell 1.1");
var_Items->InsertItem(h,vtMissing,"Cell 1.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("R2");
var_Items->InsertItem(h,vtMissing,"Cell 2.1");
var_Items->InsertItem(h,vtMissing,"Cell 2.2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spTree1->EndUpdate();
|
451
|
Can I display the cell's check box after the text
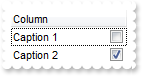
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Column")));
var_Column->PutDef(EXTREELib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutDef(EXTREELib::exCellDrawPartsOrder,"caption,check");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->PutCellHasCheckBox(var_Items->AddItem("Caption 1"),long(0),VARIANT_TRUE);
var_Items->PutCellHasCheckBox(var_Items->AddItem("Caption 2"),long(0),VARIANT_TRUE);
|
450
|
Can I change the order of the parts in the cell, as checkbox after the text, and so on
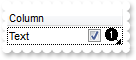
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Column")))->PutDef(EXTREELib::exCellDrawPartsOrder,"caption,check,icon,icons,picture");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Text");
var_Items->PutCellImage(h,long(0),1);
var_Items->PutCellHasCheckBox(h,long(0),VARIANT_TRUE);
|
449
|
Can I have an image displayed after the text. Can I get that effect without using HTML content
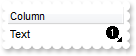
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Column")))->PutDef(EXTREELib::exCellDrawPartsOrder,"caption,icon,check,icons,picture");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Text");
var_Items->PutCellImage(h,long(0),1);
|
448
|
Does your control support RightToLeft property for RTL languages or right to left
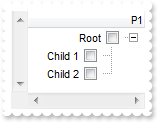
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutScrollBars(EXTREELib::exDisableBoth);
spTree1->PutLinesAtRoot(EXTREELib::exLinesAtRoot);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"P1")));
var_Column->PutDef(EXTREELib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutPartialCheck(VARIANT_TRUE);
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spTree1->PutRightToLeft(VARIANT_TRUE);
spTree1->EndUpdate();
|
447
|
Is there any way to display the vertical scroll bar on the left side, as I want to align my data to the right

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutScrollBars(EXTREELib::exDisableBoth);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"C1");
var_Columns->Add(L"C2");
var_Columns->Add(L"C3");
var_Columns->Add(L"C4");
var_Columns->Add(L"C5");
var_Columns->Add(L"C6");
var_Columns->Add(L"C7");
var_Columns->Add(L"C8");
spTree1->PutRightToLeft(VARIANT_TRUE);
spTree1->EndUpdate();
|
446
|
How can I use the CASE statement (CASE usage)
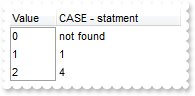
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Value")))->PutWidth(24);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"CASE - statment")));
var_Column->PutComputedField(_bstr_t("%0 case (default:'not found';1:%0;2:2*%0;3:3*%0;4:4*%0;5:5*%0;7:'Seven';8:'Eight';9:'Nine';11:'Eleven';13:'Thirtheen';14:'Fourt") +
"heen')");
var_Column->PutToolTip(var_Column->GetComputedField());
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
spTree1->EndUpdate();
|
445
|
How can I use the CASE statement (CASE usage)
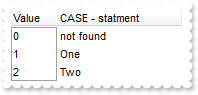
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Value")))->PutWidth(24);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"CASE - statment")));
var_Column->PutComputedField(_bstr_t("%0 case (default:'not found';1:'One';2:'Two';3:'Three';4:'Four';5:'Five';7:'Seven';8:'Eight';9:'Nine';11:'Eleven';13:'Thirtheen") +
"';14:'Fourtheen')");
var_Column->PutToolTip(var_Column->GetComputedField());
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
spTree1->EndUpdate();
|
444
|
I have seen the IN function but it returns -1 or 0. How can I display the value being found ( SWITCH usage )
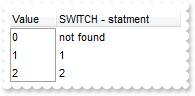
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Value")))->PutWidth(24);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"SWITCH - statment")));
var_Column->PutComputedField(L"%0 switch ('not found', 1,2,3,4,5,7,8,9,11,13,14)");
var_Column->PutToolTip(var_Column->GetComputedField());
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
spTree1->EndUpdate();
|
443
|
I have a large collection of constant values and using or operator is a time consuming (IN usage). Is there any way to increase the speed to check if a value maches the collection
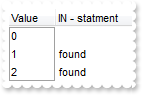
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Value")))->PutWidth(24);
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"IN - statment")));
var_Column->PutComputedField(L"%0 in (1,2,3,4,5,7,8,9,11,13,14) ? 'found' : ''");
var_Column->PutToolTip(var_Column->GetComputedField());
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
spTree1->EndUpdate();
|
442
|
Is is possible to use HTML tags to display in the filter caption
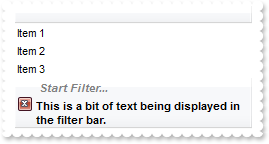
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarCaption(L"This is a bit of text being displayed in the filter bar.");
spTree1->GetColumns()->Add(L"");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
spTree1->EndUpdate();
|
441
|
How can I find the number of items after filtering
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->GetColumns()->Add(L"");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("");
var_Items->PutCellCaption(h,long(0),var_Items->GetVisibleItemCount());
spTree1->EndUpdate();
|
440
|
How can I change the filter caption
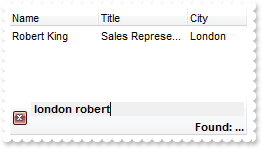
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::FilterPromptEnum(EXTREELib::exFilterPromptWords | EXTREELib::exFilterPromptContainsAll));
spTree1->PutFilterBarPromptPattern(L"london robert");
spTree1->PutFilterBarCaption(L"<r>Found: ... ");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
439
|
While using the filter prompt is it is possible to use wild characters
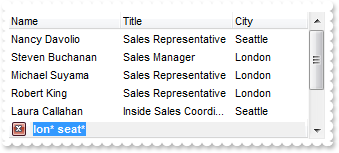
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptPattern);
spTree1->PutFilterBarPromptPattern(L"lon* seat*");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
438
|
How can I list all items that contains any of specified words, not necessary at the beggining
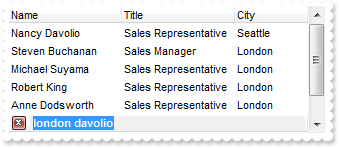
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::FilterPromptEnum(EXTREELib::exFilterPromptStartWords | EXTREELib::exFilterPromptContainsAny));
spTree1->PutFilterBarPromptPattern(L"london davolio");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
437
|
How can I list all items that contains any of specified words, not strings
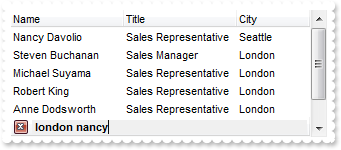
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::FilterPromptEnum(EXTREELib::exFilterPromptWords | EXTREELib::exFilterPromptContainsAny));
spTree1->PutFilterBarPromptPattern(L"london nancy");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
436
|
How can I list all items that contains all specified words, not strings
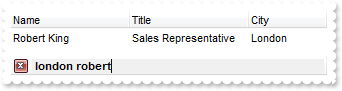
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::FilterPromptEnum(EXTREELib::exFilterPromptWords | EXTREELib::exFilterPromptContainsAll));
spTree1->PutFilterBarPromptPattern(L"london robert");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
435
|
I've noticed that the filtering by prompt is not case sensitive, is is possible to make it case sensitive
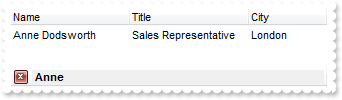
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::FilterPromptEnum(EXTREELib::exFilterPromptCaseSensitive | EXTREELib::exFilterPromptContainsAny));
spTree1->PutFilterBarPromptPattern(L"Anne");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
434
|
Is it possible to list only items that ends with any of specified strings
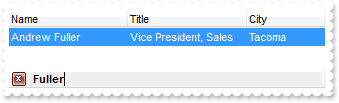
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptEndWith);
spTree1->PutFilterBarPromptColumns("0");
spTree1->PutFilterBarPromptPattern(L"Fuller");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
433
|
Is it possible to list only items that ends with any of specified strings
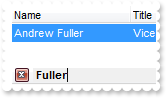
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptEndWith);
spTree1->PutFilterBarPromptColumns("0");
spTree1->PutFilterBarPromptPattern(L"Fuller");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
432
|
Is it possible to list only items that starts with any of specified strings
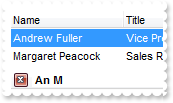
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptStartWith);
spTree1->PutFilterBarPromptColumns("0");
spTree1->PutFilterBarPromptPattern(L"An M");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
431
|
Is it possible to list only items that starts with specified string
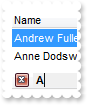
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptStartWith);
spTree1->PutFilterBarPromptColumns("0");
spTree1->PutFilterBarPromptPattern(L"A");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
430
|
How can I specify that the list should include any of the seqeunces in the pattern
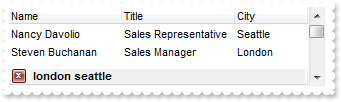
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptContainsAny);
spTree1->PutFilterBarPromptPattern(L"london seattle");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
429
|
How can I specify that all sequences in the filter pattern must be included in the list
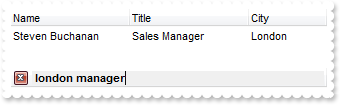
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptType(EXTREELib::exFilterPromptContainsAll);
spTree1->PutFilterBarPromptPattern(L"london manager");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
428
|
How do I change at runtime the filter prompt
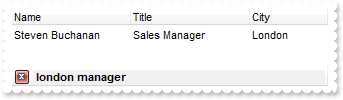
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptPattern(L"london manager");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
427
|
How do I specify to filter only a single column when using the filter prompt
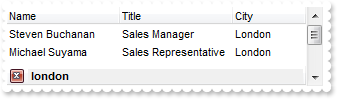
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPromptColumns("2,3");
spTree1->PutFilterBarPromptPattern(L"london");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
426
|
How do I change the prompt or the caption being displayed in the filter bar

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
spTree1->PutFilterBarPrompt(L"changed");
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
spTree1->EndUpdate();
|
425
|
How do I enable the filter prompt feature
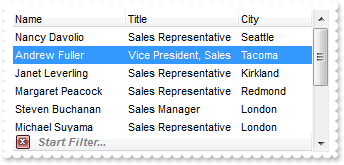
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->BeginUpdate();
spTree1->PutColumnAutoResize(VARIANT_TRUE);
spTree1->PutContinueColumnScroll(VARIANT_FALSE);
spTree1->PutMarkSearchColumn(VARIANT_FALSE);
spTree1->PutSearchColumnIndex(1);
spTree1->PutFilterBarPromptVisible(VARIANT_TRUE);
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellCaption(h0,long(1),"Vice President, Sales");
var_Items->PutCellCaption(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellCaption(h0,long(1),"Sales Manager");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellCaption(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellCaption(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellCaption(h0,long(1),"Sales Representative");
var_Items->PutCellCaption(h0,long(2),"London");
spTree1->EndUpdate();
|
424
|
I have an EBN file how can I apply different colors to it, so no need to create a new one
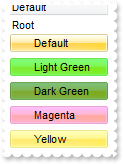
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spTree1->PutSelBackColor(spTree1->GetBackColor());
spTree1->PutSelForeColor(spTree1->GetForeColor());
spTree1->PutHasLines(EXTREELib::exNoLine);
spTree1->GetColumns()->Add(L"Default");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
long hC = var_Items->InsertItem(h,vtMissing,"Default");
var_Items->PutItemBackColor(hC,0x1000000);
var_Items->PutItemHeight(var_Items->InsertItem(h,vtMissing,""),6);
hC = var_Items->InsertItem(h,vtMissing,"Light Green");
var_Items->PutItemBackColor(hC,0x100ff00);
var_Items->PutItemHeight(var_Items->InsertItem(h,vtMissing,""),6);
hC = var_Items->InsertItem(h,vtMissing,"Dark Green");
var_Items->PutItemBackColor(hC,0x1007f00);
var_Items->PutItemHeight(var_Items->InsertItem(h,vtMissing,""),6);
hC = var_Items->InsertItem(h,vtMissing,"Magenta");
var_Items->PutItemBackColor(hC,0x1ff7fff);
var_Items->PutItemHeight(var_Items->InsertItem(h,vtMissing,""),6);
hC = var_Items->InsertItem(h,vtMissing,"Yellow");
var_Items->PutItemBackColor(hC,0x17fffff);
var_Items->PutItemHeight(var_Items->InsertItem(h,vtMissing,""),6);
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
423
|
How can I change the foreground color for a particular column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Column 1");
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Column 2")))->PutDef(EXTREELib::exHeaderForeColor,long(8439039));
var_Columns->Add(L"Column 3");
|
422
|
How can I change the background color for a particular column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnsPtr var_Columns = spTree1->GetColumns();
var_Columns->Add(L"Column 1");
((EXTREELib::IColumnPtr)(var_Columns->Add(L"Column 2")))->PutDef(EXTREELib::exHeaderBackColor,long(8439039));
var_Columns->Add(L"Column 3");
|
421
|
How can I display the column using currency format and enlarge the font for certain values
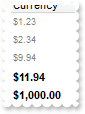
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Currency")));
var_Column->PutDef(EXTREELib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"len(value) ? ((0:=dbl(value)) < 10 ? '<fgcolor=808080><font ;7>' : '<b>') + currency(=:0)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("9.94");
var_Items->AddItem("11.94");
var_Items->AddItem("1000");
|
420
|
How can I highlight only parts of the cells

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"")));
var_Column->PutDef(EXTREELib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"value replace 'hil' with '<fgcolor=FF0000><b>hil</b></fgcolor>'");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
419
|
How can I get the number of occurrences of a specified string in the cell
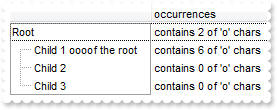
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"");
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"occurrences")));
var_Column->PutComputedField(L"lower(%0) count 'o'");
var_Column->PutFormatColumn(L"'contains ' + value + ' of \\'o\\' chars'");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1 oooof the root");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
418
|
How can I display dates in my format

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Date")));
var_Column->PutDef(EXTREELib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"'<b>' + year(0:=date(value)) + '</b><fgcolor=808080><font ;6> (' + month(=:0) + ' - ' + day(=:0) +')'");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,21,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,24,0,00,00).operator DATE());
|
417
|
How can I display dates in short format
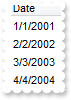
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Date")))->PutFormatColumn(L"shortdate(value)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,0,00,00).operator DATE());
|
416
|
How can I display dates in long format
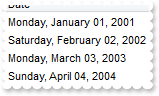
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Date")))->PutFormatColumn(L"longdate(value)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,2,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,3,0,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,4,0,00,00).operator DATE());
|
415
|
How can I display only the right part of the cell
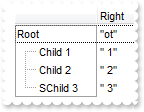
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"");
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Right")));
var_Column->PutComputedField(L"%0 right 2");
var_Column->PutFormatColumn(L"'\"' + value + '\"'");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"SChild 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
414
|
How can I display only the left part of the cell
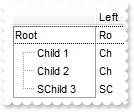
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Left")))->PutComputedField(L"%0 left 2");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"SChild 3");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
413
|
How can I display true or false instead 0 and -1

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Boolean")))->PutFormatColumn(L"value != 0 ? 'true' : 'false'");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(VARIANT_TRUE);
var_Items->AddItem(VARIANT_FALSE);
var_Items->AddItem(VARIANT_TRUE);
var_Items->AddItem(long(0));
var_Items->AddItem(long(1));
|
412
|
How can I display icons or images instead numbers
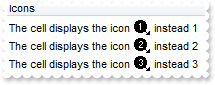
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
EXTREELib::IColumnPtr var_Column = ((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Icons")));
var_Column->PutDef(EXTREELib::exCellCaptionFormat,long(1));
var_Column->PutFormatColumn(L"'The cell displays the icon <img>'+value+'</img> instead ' + value");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(long(1));
var_Items->AddItem(long(2));
var_Items->AddItem(long(3));
|
411
|
How can I display the column using currency
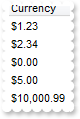
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Currency")))->PutFormatColumn(L"currency(dbl(value))");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("0");
var_Items->AddItem(long(5));
var_Items->AddItem("10000.99");
|
410
|
How can I display the currency only for not empty cells
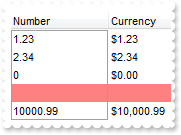
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Number");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Currency")))->PutComputedField(L"len(%0) ? currency(dbl(%0)) : ''");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem("1.23");
var_Items->AddItem("2.34");
var_Items->AddItem("0");
var_Items->PutItemBackColor(var_Items->AddItem(vtMissing),RGB(255,128,128));
var_Items->AddItem("10000.99");
|
409
|
Is there a function to display the number of days between two date including the number of hours
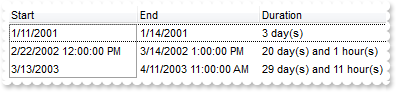
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Start")))->PutWidth(32);
spTree1->GetColumns()->Add(L"End");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Duration")))->PutComputedField(_bstr_t("2:=((1:=int(0:= date(%1)-date(%0))) = 0 ? '' : str(=:1) + ' day(s)') + ( 3:=round(24*(=:0-floor(=:0))) ? (len(=:2) ? ' and ' : ") +
"'') + =:3 + ' hour(s)' : '' )");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem(COleDateTime(2001,1,11,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,14,0,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2002,2,22,12,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2002,3,14,13,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2003,4,11,11,00,00).operator DATE());
|
408
|
Is there a function to display the number of days between two date including the number of hours
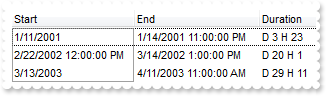
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Start");
spTree1->GetColumns()->Add(L"End");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Duration")))->PutComputedField(L"\"D \" + int(date(%1)-date(%0)) + \" H \" + round(24*(date(%1)-date(%0) - floor(date(%1)-date(%0))))");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem(COleDateTime(2001,1,11,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,14,23,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2002,2,22,12,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2002,3,14,13,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2003,4,11,11,00,00).operator DATE());
|
407
|
How can I display the number of days between two dates
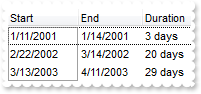
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Start");
spTree1->GetColumns()->Add(L"End");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Duration")))->PutComputedField(L"(date(%1)-date(%0)) + ' days'");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
long h = var_Items->AddItem(COleDateTime(2001,1,11,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2001,1,14,0,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2002,2,22,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2002,3,14,0,00,00).operator DATE());
h = var_Items->AddItem(COleDateTime(2003,3,13,0,00,00).operator DATE());
var_Items->PutCellCaption(h,long(1),COleDateTime(2003,4,11,0,00,00).operator DATE());
|
406
|
How can I get second part of the date
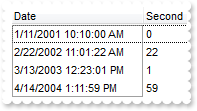
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Date");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Second")))->PutComputedField(L"sec(date(%0))");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,10,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,01,22).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,23,01).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,11,59).operator DATE());
|
405
|
How can I get minute part of the date
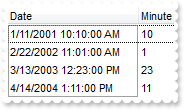
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Date");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Minute")))->PutComputedField(L"min(date(%0))");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,10,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,01,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,23,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,11,00).operator DATE());
|
404
|
How can I check the hour part only so I know it was afternoon
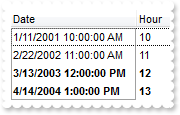
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetConditionalFormats()->Add(L"hour(%0)>=12",vtMissing)->PutBold(VARIANT_TRUE);
spTree1->GetColumns()->Add(L"Date");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Hour")))->PutComputedField(L"hour(%0)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
403
|
What about a function to get the day in the week, or days since Sunday
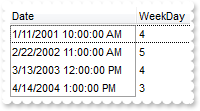
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Date");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"WeekDay")))->PutComputedField(L"weekday(%0)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
402
|
Is there any function to get the day of the year or number of days since January 1st
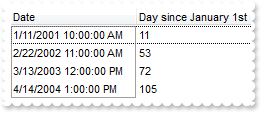
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Date");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Day since January 1st")))->PutComputedField(L"yearday(%0)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|
401
|
How can I display only the day of the date
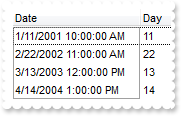
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXTREELib' for the library: 'ExTree 1.0 Control Library'
#import <ExTree.dll>
using namespace EXTREELib;
*/
EXTREELib::ITreePtr spTree1 = GetDlgItem(IDC_TREE1)->GetControlUnknown();
spTree1->GetColumns()->Add(L"Date");
((EXTREELib::IColumnPtr)(spTree1->GetColumns()->Add(L"Day")))->PutComputedField(L"day(%0)");
EXTREELib::IItemsPtr var_Items = spTree1->GetItems();
var_Items->AddItem(COleDateTime(2001,1,11,10,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2002,2,22,11,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2003,3,13,12,00,00).operator DATE());
var_Items->AddItem(COleDateTime(2004,4,14,13,00,00).operator DATE());
|